c基础
First Post:
Last Update:
Word Count:
Read Time:
Last Update:
Word Count:
807
Read Time:
4 min
C language
for循环
1 |
|
打开文件
1 |
|
斐波那契数列
1 |
|
金字塔
1 |
|
汉罗塔攻略
1 |
|
递归累加
递归函数来实现1+2+3+4+….+100
1 |
|
桶排序
1 |
|
宏定义
1 |
|
位域
bitfiled
1 |
|
打赏点小钱
支付宝 | Alipay
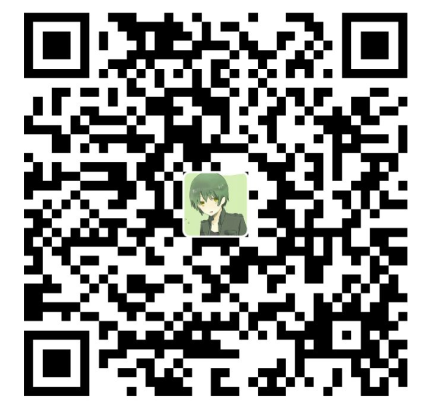
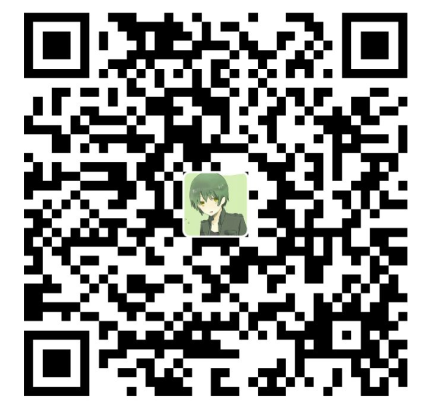
微信 | WeChat
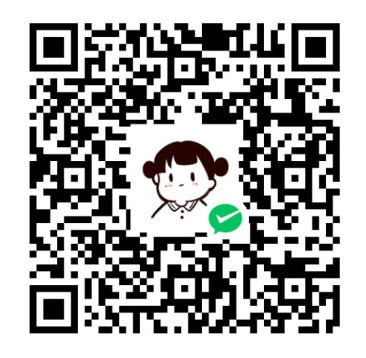
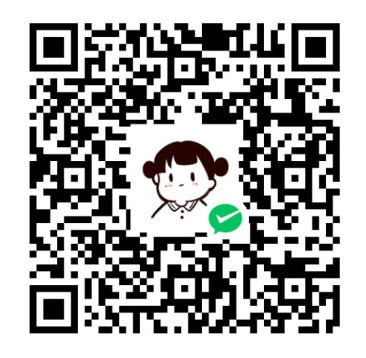
1 |
|
1 |
|
1 |
|
1 |
|
1 |
|
递归函数来实现1+2+3+4+….+100
1 |
|
1 |
|
1 |
|
bitfiled
1 |
|